A Comprehensive Guide to OWL JS Hooks
OWL (Odoo Web Library) provides a modern framework for building web components using JavaScript. Hooks in OWL JS are particularly powerful tools that improve the way developers manage state, access the DOM, and organize complex component logic. Understanding how to leverage these hooks can transform your web development workflow, especially for projects based on Odoo or similar systems. In this guide, we'll break down the essential OWL JS hooks and learn how to use them effectively.
Why Use OWL JS Hooks?
Hooks in OWL are designed to:
- Reuse Stateful Logic: Hooks allow you to reuse logic between components, making your code cleaner and more efficient.
- Organize Code Better: In complex components, hooks help structure code by feature, reducing the chance of messy code.
- Simplify State Management: Hooks let you use state in functional components without needing to write a class, thus simplifying the development process.
Note: All hooks in OWL must be called inside the setup method.
useState : Managing State in Your Components
The useState hook is fundamental for handling component state. It lets you keep track of variable changes dynamically. Simply put, it's a tool to make your component reactive.
Syntax and Usage
JavaScript
import { Component, useState } from "@odoo/owl";
class Counter extends Component {
static template = xml`
<button t-on-click="increment">
Click Me! [<t t-esc="state.value"/>]
</button>
`;
state = useState({ value: 0 });
increment() {
this.state.value++;
}
}
Explanation
- useState Initialization: The useState function initializes the state of the component. In the above example, state is an object with a value property.
- Reactivity: Every time this.state.value changes, the component re-renders, reflecting the updated state.
- Event Handling: The increment method increases value by one each time the button is clicked.
Tip: Use useState for simple to moderately complex state logic within a component.
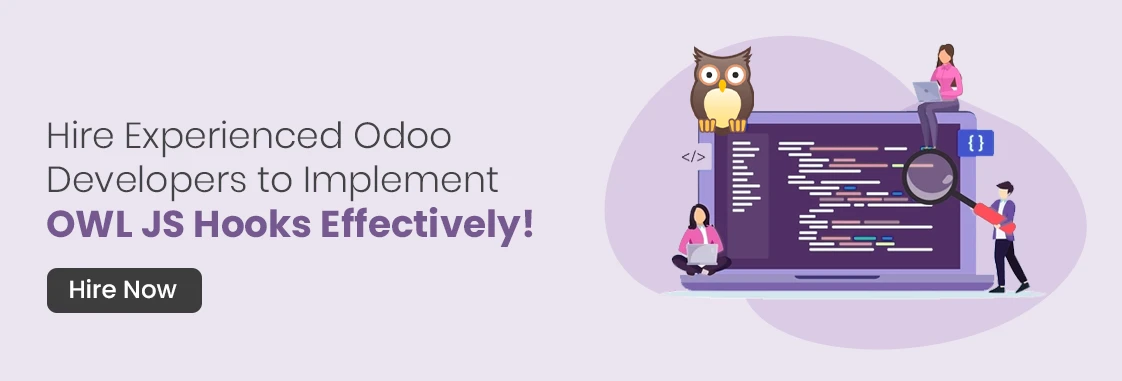
useEffect : Handling Side Effects
The useEffect hook is used to perform side effects in your components, such as fetching data, manually updating the DOM, or setting timers. It allows you to run specific code blocks based on changes in your component or certain variables.
Basic Example
JavaScript
import { Component, useEffect } from "@odoo/owl";
// Executes only when the component mounts (renders for the first time).
useEffect(
() => {
// Logic to execute, such as fetching data or logging
},
() => []
);
Dependency-Based Example
JavaScript
import { useState, Component } from “@odoo/owl”;
class Counter extends Component {
setup() {
const count = useState({ count: 0 });
useEffect(
() => {
// Execute logic whenever 'count' changes
},
() => [count]
);
}
}
- Dependency Array: If the dependency array is empty ('[]'), the effect runs only when the component is mounted. If you include variables (like count), the effect runs whenever these variables change.
- Common Use Cases:
- Fetching data from an API
- Listening to global events and cleaning up afterward
- Running animations based on state
Best Practice: Always manage cleanup inside useEffect to prevent memory leaks, especially for event listeners or intervals.
useRef: Accessing and Managing DOM Elements
useRef is a powerful hook that returns a reference to a DOM element, allowing you to perform operations directly on it. This hook is particularly useful for manipulating or focusing elements.
Example Usage
JavaScript
<div>
<input t-ref="someInput" />
<span>Hello</span>
</div>
import { useState, Component } from “@odoo/owl”;
class Parent extends Component {
inputRef = useRef("someInput");
someMethod() {
// If the component is mounted, refs are active:
// this.inputRef.el is the input HTML element
}
}
Explanation
- Referencing Elements: In this example, inputRef points to the input element. You can access the HTML element through this.inputRef.el.
- Common Scenarios:
- Programmatically focusing an input field
- Manipulating the DOM directly, such as setting element attributes or styles
- Managing third-party libraries that require a direct reference to DOM nodes
useService: Integrating External Services
The useService hook is used to inject and use external services within your components. In OWL, services are singleton objects that manage global state or provide functionality like API communication, notification handling, or data caching.
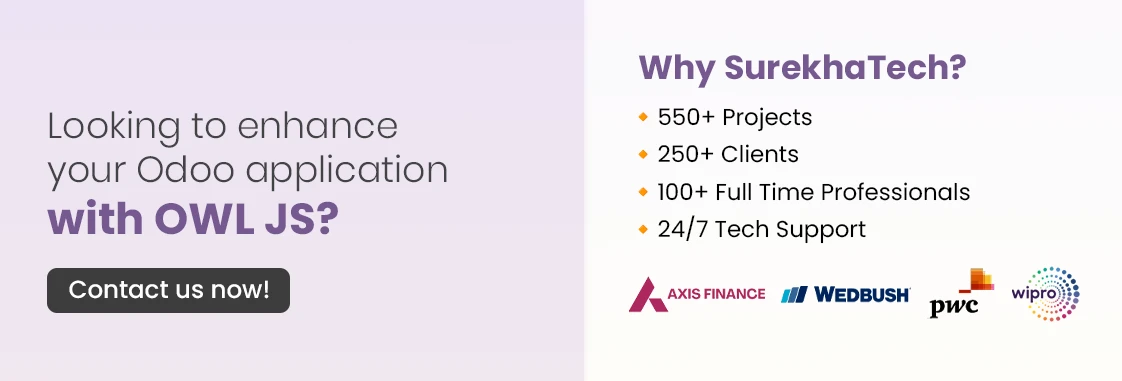
Example Usage
Assuming you have a NotificationService that you want to use in your component:
JavaScript
import { useState, Component } from “@odoo/owl”;
import { useService } from "@web/core/utils/hooks";
class NotificationComponent extends Component {
setup() {
const notificationService = useService("notification");
function sendNotification() {
notificationService.notify("This is a test notification!");
}
return { sendNotification };
}
}
Explanation
- Service Injection: The useService hook allows you to access and use a global service defined in your application.
- Global State Management: Services are particularly useful for handling state or logic that needs to be shared across multiple components.
- Use Cases:
- Managing user authentication state
- Centralized logging or error reporting
- Communication between non-hierarchical components
Conclusion
OWL JS hooks, like useState,useEffect,useRef,useService, provide a clean and efficient way to handle state, side effects, DOM interactions, and external services in your components. By leveraging these hooks, you can write more structured, readable, and maintainable code. When combined with Odoo development services, these capabilities enable seamless customization and optimization of Odoo applications, ensuring better performance and scalability. As you continue working with OWL, experimenting with these hooks will help you refine your component structure for improved reusability and efficiency.